Create a Nibless iOS App
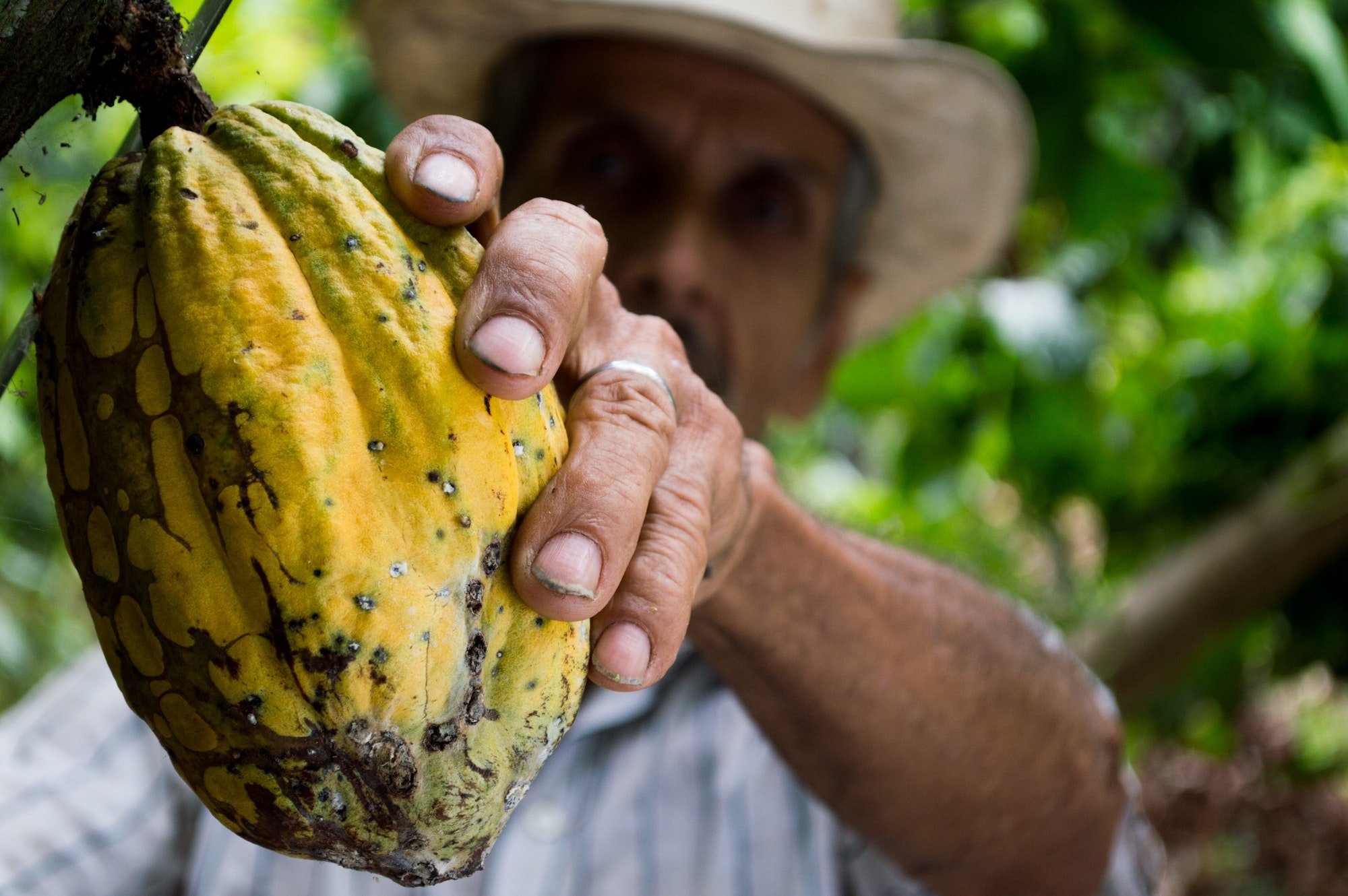
I found that I prefer to create iOS apps completely in code and without any storyboards or nibs (née nibless). In this article, I'd like to outline the process I use to do this for the benefit of others who prefer a pure code approach as well.
First, remove the following two entries from your app's Info.plist. References are given at the bottom so you can know what you are deleting.
Information Property List
├─ Application Scene Manifest
│ ╰─ Scene Configuration
│ ╰─ Application Session Role
│ ╰─ Item 0
│ ╰─ Storyboard Name* (1)
╰─ Main storyboard file base name* (2)
* Remove this entry
Then, you can go ahead and delete the Main.storyboard file from your project.
Next, update your AppDelegate.swift to look like the following.
import UIKit
@main
final class AppDelegate: UIResponder, UIApplicationDelegate {
func application(
_ application: UIApplication,
configurationForConnecting session: UISceneSession,
options: UIScene.ConnectionOptions
) -> UISceneConfiguration {
let sceneConfiguration = UISceneConfiguration(
name: "Default Configuration",
sessionRole: session.role
)
return sceneConfiguration
}
}
Lastly, update your SceneDelegate.swift to look like this.
import UIKit
final class SceneDelegate: UIResponder, UIWindowSceneDelegate {
var window: UIWindow?
func scene(
_ scene: UIScene,
willConnectTo session: UISceneSession,
options connectionOptions: UIScene.ConnectionOptions
) {
guard let windowScene = (scene as? UIWindowScene) else {
return
}
let navigationController = UINavigationController(
rootViewController: ViewController()
)
window = UIWindow(windowScene: windowScene)
window?.rootViewController = navigationController
window?.makeKeyAndVisible()
}
}
If you like this approach, you could even take it a step further and create a project template with this code in there. Here↗︎ is a relatively recent example that you can follow to create a nibless project template. I don't use project templates because I don't rely on undocumented behavior, so I just use the steps outlined above.
Sources
- Info.plist: UISceneStoryboardFile↗︎
- Info.plist: UIMainStoryboardFile↗︎